Example: 1
Step #1:
yarn add firebase...................................................................................................................................................................................................................................
Step #2:
import React from 'react'
import firebase from 'firebase/app'
import 'firebase/auth'
import 'firebase/firestore'
const config = {
apiKey: "AIzaSyCeLflgDuxPKoLW6Ch9JfP2pC5H6PFJ7wE",
authDomain: "fir-auth-25bb5.firebaseapp.com",
databaseURL: "https://fir-auth-25bb5.firebaseio.com",
projectId: "fir-auth-25bb5",
storageBucket: "fir-auth-25bb5.appspot.com",
messagingSenderId: "911081011536",
appId: "1:911081011536:web:23dac88c4382dbe7"
}
firebase.initializeApp(config)
const auth = firebase.auth()
const firestore = firebase.firestore()
const provider = new firebase.auth.GoogleAuthProvider()
provider.setCustomParameters({ prompt: 'select_account' })
const signInWithGoogle = ()=>auth.signInWithPopup(provider)
const App = () => {
return (
<div>
<h1>hello</h1>
<button onClick={signInWithGoogle}>signInWithGoogle</button>
</div>
)
}
export default App
...................................................................................................................................................................................................................
Example: 2 (how to get user information)
Step #1:firebase/firebase.utils
import firebase from 'firebase/app'
import 'firebase/auth'
import 'firebase/firestore'
const config = {
apiKey: "AIzaSyCeLflgDuxPKoLW6Ch9JfP2pC5H6PFJ7wE",
authDomain: "fir-auth-25bb5.firebaseapp.com",
databaseURL: "https://fir-auth-25bb5.firebaseio.com",
projectId: "fir-auth-25bb5",
storageBucket: "fir-auth-25bb5.appspot.com",
messagingSenderId: "911081011536",
appId: "1:911081011536:web:23dac88c4382dbe7"
}
firebase.initializeApp(config)
export const auth = firebase.auth()
export const firestore = firebase.firestore()
const provider = new firebase.auth.GoogleAuthProvider()
provider.setCustomParameters({ prompt: 'select_account' })
export const signInWithGoogle = ()=>auth.signInWithPopup(provider)
...................................................................................................................................................................................................................
Step #2:
App.js
import React, { Component } from 'react'
import { auth } from './firebase/firebase.utils'
import { signInWithGoogle } from './firebase/firebase.utils'
class App extends Component {
constructor(props) {
super(props)
this.state = {
currentUser: null
}
}
componentDidMount(){
auth.onAuthStateChanged(user=>{
this.setState({currentUser:user})
console.log(user)
})
}
render() {
return (
<div className='App' >
<h1>hello</h1>
<button type='button' onClick={signInWithGoogle}>signInWithGoogle</button>
</div>
)
}
}
export default App
...................................................................................................................................................................................................................
reference sites:
More details follow as below LInk
https://firebase.googleblog.com/2016/07/have-you-met-realtime-database.html
https://blog.jscrambler.com/integrating-firebase-with-react-native/?utm_source=facebook.com&utm_medium=referral&utm_campaign=group&fbclid=IwAR1zxbCH1xlrJuuUJe_W6QQ5kpsRVQocFodTFgjaPUMGlKZuuwakHg3kt4I
Happy coding :)
..................................................................................................................................................................................................................................
Example: # 3 | Firebase Notification Integration in React Native 0.60+
......................................................................................................................................................................................................................................
Step : #1
Step : #1.1 Firebase Console
https://console.firebase.google.com/?pli=1
......................................................................................................................................................................................................................................
Step : #1.2
- Enter your project name.
......................................................................................................................................................................................................................................
......................................................................................................................................................................................................................................
Step : #1.4
......................................................................................................................................................................................................................................
Step : #1.5
......................................................................................................................................................................................................................................
Step : #1.6
......................................................................................................................................................................................................................................
Step : #1.7
- You add according to your required platform.
......................................................................................................................................................................................................................................
Step : #1.8
- Where you find your package name: android/app/src/main/AndroidManifest.xml
......................................................................................................................................................................................................................................
Step : #1.9
- Download the 'google-services.json' file to the directory 'android/app', Click 'Next' and complete the process.
or
......................................................................................................................................................................................................................................
Step : #2
Step : #2.1 first we need to install this plugin.
npm install --save react-native-firebase
or
yarn add react-native-firebase
......................................................................................................................................................................................................................................
Step : #2.2
On your build.gradle file add it.
android/build.gradle
classpath 'com.google.gms:google-services:4.3.2'
On your build.gradle file add it.
android/build.gradle
classpath 'com.google.gms:google-services:4.3.2'
......................................................................................................................................................................................................................................
Step : #2.3
android/app/build.gradle add the following lines to your dependencies
implementation "com.google.android.gms:play-services-base:17.0.0" // it's not mendatory
implementation "com.google.firebase:firebase-core:17.0.1" // it's not mendatory
implementation "com.google.firebase:firebase-messaging:19.0.0" //<-- Add Here
implementation 'me.leolin:ShortcutBadger:1.1.21@aar' //<-- Add this line if you wish to use badge on Android
apply plugin: 'com.google.gms.google-services' // <-- Add one line Here and below one
Note : run the project , react-native run-android
because next process will work.
android/app/build.gradle add the following lines to your dependencies
Add the Firebase Cloud Messaging dependency and optional ShortcutBadger dependency to android/app/build.gradle
implementation 'com.google.firebase:firebase-analytics:17.2.0'
implementation "com.google.firebase:firebase-core:17.0.1" // it's not mendatory
implementation "com.google.firebase:firebase-messaging:19.0.0" //<-- Add Here
implementation 'me.leolin:ShortcutBadger:1.1.21@aar' //<-- Add this line if you wish to use badge on Android
apply plugin: 'com.google.gms.google-services' // <-- Add one line Here and below one
Note : run the project , react-native run-android
because next process will work.
......................................................................................................................................................................................................................................
Step : #2.4
android/app/src/main/java/MainApplication.java add the following lines of code.
import io.invertase.firebase.RNFirebasePackage;
import io.invertase.firebase.messaging.RNFirebaseMessagingPackage;
import io.invertase.firebase.notifications.RNFirebaseNotificationsPackage;
packages.add(new RNFirebaseMessagingPackage());
packages.add(new RNFirebaseNotificationsPackage());
android/app/src/main/java/MainApplication.java add the following lines of code.
import io.invertase.firebase.RNFirebasePackage;
import io.invertase.firebase.messaging.RNFirebaseMessagingPackage;
import io.invertase.firebase.notifications.RNFirebaseNotificationsPackage;
packages.add(new RNFirebaseMessagingPackage());
packages.add(new RNFirebaseNotificationsPackage());
......................................................................................................................................................................................................................................
Step : #2.5
android/app/src/main/AndroidManifest.xml add the following lines of code.
android/app/src/main/AndroidManifest.xml add the following lines of code.
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
<uses-permission android:name="android.permission.VIBRATE" />
<!-- start of firebase messaging services -->
<service android:name="io.invertase.firebase.messaging.RNFirebaseMessagingService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
<!-- Background Messages (Optional) -->
<service android:name="io.invertase.firebase.messaging.RNFirebaseInstanceIdService">
<intent-filter>
<action android:name="com.google.firebase.INSTANCE_ID_EVENT"/>
</intent-filter>
</service>
....................................................................................................................................................................................................................................
Step : #2.6
App.js
import React, { Component } from 'react'
import { View, Text } from 'react-native'
import firebase from 'react-native-firebase';
class App extends Component {
async getToken() {
let fcmToken = await AsyncStorage.getItem('fcmToken');
if (!fcmToken) {
fcmToken = await firebase.messaging().getToken();
if (fcmToken) {
await AsyncStorage.setItem('fcmToken', fcmToken);
}
}
}
async checkPermission() {
const enabled = await firebase.messaging().hasPermission();
if (enabled) {
this.getToken();
} else {
this.requestPermission();
}
}
async requestPermission() {
try {
await firebase.messaging().requestPermission();
this.getToken();
} catch (error) {
console.log('permission rejected');
}
}
async createNotificationListeners() {
firebase.notifications().onNotification(notification => {
notification.android.setChannelId('insider').setSound('default')
firebase.notifications().displayNotification(notification)
});
}
componentDidMount() {
const channel = new firebase.notifications.Android.Channel('insider', 'insider channel', firebase.notifications.Android.Importance.Max)
firebase.notifications().android.createChannel(channel);
this.checkPermission();
this.createNotificationListeners();
}
render() {
return (
<>
</>
)
}
}
export default App
import React, { Component } from 'react'
import { View, Text } from 'react-native'
import firebase from 'react-native-firebase';
class App extends Component {
async getToken() {
let fcmToken = await AsyncStorage.getItem('fcmToken');
if (!fcmToken) {
fcmToken = await firebase.messaging().getToken();
if (fcmToken) {
await AsyncStorage.setItem('fcmToken', fcmToken);
}
}
}
async checkPermission() {
const enabled = await firebase.messaging().hasPermission();
if (enabled) {
this.getToken();
} else {
this.requestPermission();
}
}
async requestPermission() {
try {
await firebase.messaging().requestPermission();
this.getToken();
} catch (error) {
console.log('permission rejected');
}
}
async createNotificationListeners() {
firebase.notifications().onNotification(notification => {
notification.android.setChannelId('insider').setSound('default')
firebase.notifications().displayNotification(notification)
});
}
componentDidMount() {
const channel = new firebase.notifications.Android.Channel('insider', 'insider channel', firebase.notifications.Android.Importance.Max)
firebase.notifications().android.createChannel(channel);
this.checkPermission();
this.createNotificationListeners();
}
render() {
return (
<>
</>
)
}
}
export default App
......................................................................................................................................................................................................................................
Step : #3
Step : #3.1
Step : #3.1
......................................................................................................................................................................................................................................
Step : #3.2
......................................................................................................................................................................................................................................
Step : #3.3
......................................................................................................................................................................................................................................
......................................................................................................................................................................................................................................
Step : #3.6
......................................................................................................................................................................................................................................
Step : #3.7
keep learning :)
keep learning :)
......................................................................................................................................................................................................................................
Example : #4 [ Integrate Google Login in React Native (Android) apps with Firebase ] 0.60+
In this post, we’ll learn how to setup Google Login in React Native apps using Firebase. We’ll implement the authentication using react-native-google-signin npm package, and then test it on Android.
Install package
Create and attach new app to the project, Now create a new Android app in the project .
by default in my case is Android
create a new project using below command
react-native init googlesigninviafirebase
>cd googlesigninviafirebase
googlesigninviafirebase> yarn add react-native-google-signin
googlesigninviafirebase> yarn add react-native-firebase
Step : #4.2 Create Firebase Project
create a new Firebase project by going to Firebase Console. Give your project a name and that’s it !
https://console.firebase.google.com/
- Just mention the correct package name of your app in the Android package name input.
- Generate the key using following command
keytool -exportcert -keystore <<keystore file location>> -list -v
React Native automatically generates a debug.keystore for your Android project, which resides in Android project folder
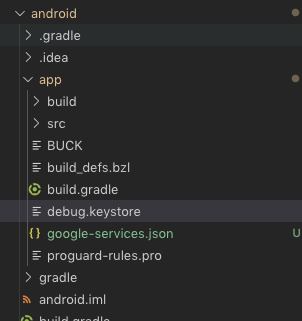
Tips: - try to paste line
Project directory >keytool -exportcert -keystore it's android folder inside app inside debug.keystore -list -v
password - android
like that in mycase.
android → app → build.gradle → signingConfigs
Enter the correct values insigningConfigs for your own key
signingConfigs {
debug {
storeFile file('debug.keystore')
storePassword 'android'
keyAlias 'androiddebugkey'
keyPassword 'android'
}
}
- Add the SHA key and package name of your project in th Firebase Console
- Next step, download the google-services.json file and put it in the android/app folder of your project.
- Update android/build.gradle with latest versions. My configuration is looks like this
classpath 'com.google.gms:google-services:4.3.2'
- Update android/app/build.gradle dependencies. My configuration is looks like this
implementation 'com.google.firebase:firebase-analytics:17.2.0'
apply plugin: 'com.google.gms.google-services'
- Run the project
Step : #4.3 Enable Google Login in Firebase
- Authentication → Sign-In Method → Google
- and click the Enable toggle.
Step : #4.4 CODE
import React, { Component } from 'react'
import { StyleSheet, View, Text, StatusBar, Image, Button } from 'react-native'
import { GoogleSignin, GoogleSigninButton, statusCodes } from 'react-native-google-signin'
export default class HomeScreen extends Component {
state = {
userDetails: {},
GoogleLogin: false,
}
render() {
return (
<View style={styles.container}>
<View style={this.state.GoogleLogin ? { display: "none" } : styles.signinContainer}>
<GoogleSigninButton
style={{ width: 192, height: 50 }}
size={GoogleSigninButton.Size.Wide}
color={GoogleSigninButton.Color.Dark}
onPress={this.signIn}>
</GoogleSigninButton>
</View>
<View style={this.state.GoogleLogin ? styles.userDetailContainer : { display: 'none' }}>
<Image style={styles.userImage} source={{ uri: this.state.userDetails.photo }}></Image>
<Text style={styles.txtEmail}>{this.state.userDetails.email}</Text>
<Text style={styles.txtName}>{this.state.userDetails.name}</Text>
<Button color="#FF5722" title='Logout' onPress={this.signOut}></Button>
</View>
</View>
)
}
signOut = async () => {
try {
await GoogleSignin.revokeAccess();
await GoogleSignin.signOut()
this.setState({
userDetails: '',
GoogleLogin: false,
}
)
} catch (error) {
console.log(error.toString())
}
}
signIn = async () => {
try {
await GoogleSignin.hasPlayServices();
const userInfo = await GoogleSignin.signIn();
console.log('User Details', JSON.stringify(userInfo))
this.setState({
GoogleLogin: true,
userDetails: userInfo.user,
})
} catch (error) {
this.setState({
GoogleLogin: false,
})
if (error.code === statusCodes.SIGN_IN_CANCELLED) {
console.log("Cancel ", statusCodes.SIGN_IN_CANCELLED)
// user cancelled the login flow
} else if (error.code === statusCodes.IN_PROGRESS) {
console.log("InProgress ", statusCodes.IN_PROGRESS)
// operation (f.e. sign in) is in progress already
} else if (error.code === statusCodes.PLAY_SERVICES_NOT_AVAILABLE) {
console.log("Not Available ", statusCodes.PLAY_SERVICES_NOT_AVAILABLE)
// play services not available or outdated
} else {
console.log("Error ", error)
}
}
};
}
GoogleSignin.configure({
scopes: ['https://www.googleapis.com/auth/drive.readonly'], // what API you want to access on behalf of the user, default is email and profile
webClientId: '956698004256-cg3e03phcd6ddpdgfcf1en0cq9o7lkni.apps.googleusercontent.com',
// client ID of type WEB for your server (needed to verify user ID and offline access)
offlineAccess: true, // if you want to access Google API on behalf of the user FROM YOUR SERVER
hostedDomain: '', // specifies a hosted domain restriction
loginHint: '', // [iOS] The user's ID, or email address, to be prefilled in the authentication UI if possible. [See docs here](https://developers.google.com/identity/sign-in/ios/api/interface_g_i_d_sign_in.html#a0a68c7504c31ab0b728432565f6e33fd)
forceConsentPrompt: true, // [Android] if you want to show the authorization prompt at each login.
accountName: '', // [Android] specifies an account name on the device that should be used
// iosClientId: '<FROM DEVELOPER CONSOLE>', // [iOS] optional, if you want to specify the client ID of type iOS (otherwise, it is taken from GoogleService-Info.plist)
});
const styles = StyleSheet.create({
container: {
flex: 1
},
signinContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
userDetailContainer: {
flex: 1,
width: '100%',
alignItems: 'center',
justifyContent: 'center'
},
userImage: {
width: 100,
height: 100,
},
txtEmail: {
color: 'black',
marginTop: 10,
fontSize: 13,
},
txtName: {
color: 'black',
margin: 10,
fontSize: 13,
}
})
Happy coding :)
......................................................................................................................................................................................................................................
Step : #4.5 ERROR
solutions -
Steps to fix:
-Go to console.developer.google.com
-Select the project.
-Go to Credentials.
-Switch to O Auth Consent screen.
-Change the app name and fill email id (optional)
-Save at the bottom
-Try logging in now and it should work.
Step : #4.8
Step : #4.9
Step : #4.10
Step : #4.11
Step : #4.12
Step : #4.13
Step : #4.14
Step : #4.15
keep learning :)
......................................................................................................................................................................................................................................
Example : #5 [ React Native Firebase Integration For Android ]
ddds
yarn add react-native-firebase
Code
import React, { Component } from 'react'
import { View, Text, Button, StyleSheet } from 'react-native'
import firebase from 'react-native-firebase'
class App extends Component {
_onSubmit = () => {
firebase.auth().createUserWithEmailAndPassword("testtest@gmail.com", "123456")
.then(user => {
alert(JSON.stringify(user))
}).catch(error => {
alert(error)
})
}
render() {
return (
<View style={styles.container}>
<Text> Hello</Text>
<Button title='Alert' onPress={this._onSubmit}> </Button>
</View>
)
}
}
export default App
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
...............................................................................................................................................................................................................................
Database Connection
implementation "com.google.firebase:firebase-database:17.0.0"
import io.invertase.firebase.database.RNFirebaseDatabasePackage;
packages.add(new RNFirebaseDatabasePackage());
import React, { Component } from 'react'
import { View, Text, Button, StyleSheet } from 'react-native'
import firebase from 'react-native-firebase'
class App extends Component {
_onUpdate = () => {
const dbRef = firebase.database().ref("testData");
dbRef.set("This is First project")
}
render() {
return (
<View style={styles.container}>
<Text> Hello</Text>
<Button title='update' onPress={this._onUpdate}> </Button>
</View>
)
}
}
export default App
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
ddds
yarn add react-native-firebase
Code
import React, { Component } from 'react'
import { View, Text, Button, StyleSheet } from 'react-native'
import firebase from 'react-native-firebase'
class App extends Component {
_onSubmit = () => {
firebase.auth().createUserWithEmailAndPassword("testtest@gmail.com", "123456")
.then(user => {
alert(JSON.stringify(user))
}).catch(error => {
alert(error)
})
}
render() {
return (
<View style={styles.container}>
<Text> Hello</Text>
<Button title='Alert' onPress={this._onSubmit}> </Button>
</View>
)
}
}
export default App
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
...............................................................................................................................................................................................................................
Database Connection
implementation "com.google.firebase:firebase-database:17.0.0"
import io.invertase.firebase.database.RNFirebaseDatabasePackage;
packages.add(new RNFirebaseDatabasePackage());
import { View, Text, Button, StyleSheet } from 'react-native'
import firebase from 'react-native-firebase'
class App extends Component {
_onUpdate = () => {
const dbRef = firebase.database().ref("testData");
dbRef.set("This is First project")
}
render() {
return (
<View style={styles.container}>
<Text> Hello</Text>
<Button title='update' onPress={this._onUpdate}> </Button>
</View>
)
}
}
export default App
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}
})
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
...............................................................................................................................................................................................................................
Project with path ':react-native-firebase' could not be found in project ':app'. #2171
solutions -
//add these lines in the android/settings.gradle
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
include ':app'
keep learning :)
yarn add react-native-firebase
setting.gradle
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
include ':app'
android\app\build.gradle
// Firebase Dependence
implementation "com.google.android.gms:play-services-base:16.1.0"
implementation "com.google.firebase:firebase-core:16.0.9"
implementation "com.google.firebase:firebase-auth:17.0.0"
// Firebase Dependence
apply plugin: 'com.google.gms.google-services'
..........................
build.gradle
classpath 'com.google.gms:google-services:4.3.2'
.................
MainApplication.js
import io.invertase.firebase.auth.RNFirebaseAuthPackage; // <-- Add this line
packages.add(new RNFirebaseAuthPackage());
......................................................................................................................................................................................................................................
Example : #6
android/app/build.gradle
solutions -
delete it implementation project(':react-native-firebase')
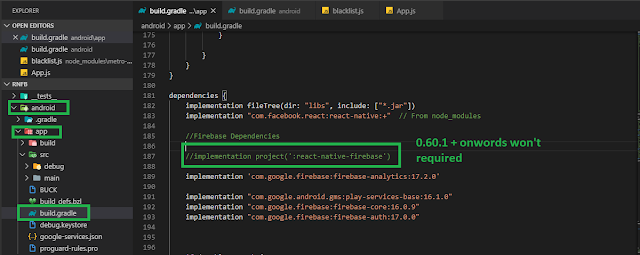
Note
Setting.gradle
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
include ':app'
or
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
Keep learning :)
solutions -
delete it implementation project(':react-native-firebase')
Setting.gradle
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
include ':app'
or
include ':react-native-firebase'
project(':react-native-firebase').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-firebase/android')
or
react-native link react-native-firebase
then
react-native unlink react-native-firebase
TIPS - do not comment it like // or // implementation project(':react-native-firebase')
got Error
Final step
android\build.gradle
//Firebase Dependencies
classpath 'com.google.gms:google-services:4.3.2'
android\app\build.gradle
//Firebase Dependencies
implementation 'com.google.firebase:firebase-analytics:17.2.0'
implementation "com.google.android.gms:play-services-base:16.1.0"
implementation "com.google.firebase:firebase-core:16.0.9"
implementation "com.google.firebase:firebase-auth:17.0.0"
apply plugin: 'com.google.gms.google-services'
......................................................................................................................................................................................................................................
Example : #7 [ React Redux Firebase Authentication web based]
config\firebase_config.js
import * as Firebase from 'firebase/app'
import 'firebase/auth'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
Firebase.initializeApp(firebaseConfig)
export const auth = Firebase.auth()
export default Firebase
SignIn
import React, { useState } from 'react'
import { auth } from '../config/firebase_config'
const SignIn = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const handleSingIn = (e) => {
e.preventDefault()
auth.signInWithEmailAndPassword(email, password)
.then((user) => {
console.log(user)
})
.catch(error => console.log(error))
}
return (
<>
<h1>Sign In</h1>
<form>
<input type='text' placeholder=' Enter Email ' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder=' Enter Password' value={password} onChange={(e) => setPassword(e.target.value)} />
</form>
<button onClick={handleSingIn}> Sign In</button>
</>
)
}
export default SignIn
SignUp
import React, { useState } from 'react'
import { auth } from '../config/firebase_config'
const SignUp = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [confirmpassword, setconfrimPassword] = useState('')
const handleSignup=(e)=>{
e.preventDefault()
if(password === confirmpassword){
auth.createUserWithEmailAndPassword(email, password)
.then(user=>console.log(user))
.catch(error=>console.log(error))
}else{
alert('password do not match')
}}
return (
<>
<h1>SignUp </h1>
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e)=>setEmail(e.target.value)}/>
<input type='password' placeholder='Enter password' value={password} onChange={(e)=>setPassword(e.target.value)}/>
<input type ='password' placeholder='Enter confirm password' value={confirmpassword} onChange={(e)=> setconfrimPassword(e.target.value)}/>
<button onClick={handleSignup}>SignUp</button>
</form>
</>
)
}
export default SignUp
config\firebase_config.js
import * as Firebase from 'firebase/app'
import 'firebase/auth'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
Firebase.initializeApp(firebaseConfig)
export const auth = Firebase.auth()
export default Firebase
SignIn
import React, { useState } from 'react'
import { auth } from '../config/firebase_config'
const SignIn = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const handleSingIn = (e) => {
e.preventDefault()
auth.signInWithEmailAndPassword(email, password)
.then((user) => {
console.log(user)
})
.catch(error => console.log(error))
}
return (
<>
<h1>Sign In</h1>
<form>
<input type='text' placeholder=' Enter Email ' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder=' Enter Password' value={password} onChange={(e) => setPassword(e.target.value)} />
</form>
<button onClick={handleSingIn}> Sign In</button>
</>
)
}
export default SignIn
SignUp
import React, { useState } from 'react'
import { auth } from '../config/firebase_config'
const SignUp = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [confirmpassword, setconfrimPassword] = useState('')
const handleSignup=(e)=>{
e.preventDefault()
if(password === confirmpassword){
auth.createUserWithEmailAndPassword(email, password)
.then(user=>console.log(user))
.catch(error=>console.log(error))
}else{
alert('password do not match')
}}
return (
<>
<h1>SignUp </h1>
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e)=>setEmail(e.target.value)}/>
<input type='password' placeholder='Enter password' value={password} onChange={(e)=>setPassword(e.target.value)}/>
<input type ='password' placeholder='Enter confirm password' value={confirmpassword} onChange={(e)=> setconfrimPassword(e.target.value)}/>
<button onClick={handleSignup}>SignUp</button>
</form>
</>
)
}
export default SignUp
......................................................................................................................................................................................................................................
Example : #8 [FIREBASE AUTH using signin and signup]
import React, { Component, useState } from 'react'
import * as Firebase from 'firebase/app'
import 'firebase/auth'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
Firebase.initializeApp(firebaseConfig)
const auth = Firebase.auth()
const SignIn = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const handleSignIn = (e) => {
e.preventDefault()
auth.signInWithEmailAndPassword(email, password)
.then(user => console.log(user))
.catch(error => console.log(error))
}
return (
<>
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder='Enter password' value={password} onChange={(e) => setPassword(e.target.value)} />
<button type='submit' onClick={handleSignIn}>Sign In</button>
</form>
</>
)
}
const SignUp = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [confirmPassword, setConfirmPassword] = useState('')
const handleSignUp = (e) => {
e.preventDefault()
if (password === confirmPassword) {
auth.createUserWithEmailAndPassword(email, password)
.then(user => console.log(user))
.catch(error => console.log(error))
} else {
alert('password does not match!')
}
}
return (
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder='Enter Password' value={password} onChange={(e) => setPassword(e.target.value)} />
<input type='password' placeholder='Enter Password' value={confirmPassword} onChange={(e) => setConfirmPassword(e.target.value)} />
<button onClick={handleSignUp}> Sign Up</button>
</form>
)
}
class App extends Component {
render() {
return (
<>
<SignIn />
<SignUp />
</>
)
}
}
export default App
import React, { Component, useState } from 'react'
import * as Firebase from 'firebase/app'
import 'firebase/auth'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
Firebase.initializeApp(firebaseConfig)
const auth = Firebase.auth()
const SignIn = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const handleSignIn = (e) => {
e.preventDefault()
auth.signInWithEmailAndPassword(email, password)
.then(user => console.log(user))
.catch(error => console.log(error))
}
return (
<>
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder='Enter password' value={password} onChange={(e) => setPassword(e.target.value)} />
<button type='submit' onClick={handleSignIn}>Sign In</button>
</form>
</>
)
}
const SignUp = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [confirmPassword, setConfirmPassword] = useState('')
const handleSignUp = (e) => {
e.preventDefault()
if (password === confirmPassword) {
auth.createUserWithEmailAndPassword(email, password)
.then(user => console.log(user))
.catch(error => console.log(error))
} else {
alert('password does not match!')
}
}
return (
<form>
<input type='email' placeholder='Enter Email' value={email} onChange={(e) => setEmail(e.target.value)} />
<input type='password' placeholder='Enter Password' value={password} onChange={(e) => setPassword(e.target.value)} />
<input type='password' placeholder='Enter Password' value={confirmPassword} onChange={(e) => setConfirmPassword(e.target.value)} />
<button onClick={handleSignUp}> Sign Up</button>
</form>
)
}
class App extends Component {
render() {
return (
<>
<SignIn />
<SignUp />
</>
)
}
}
export default App
......................................................................................................................................................................................................................................
Example : #9 [ Firebase + React + crud]
import React, { Component } from 'react'
import firebase from 'firebase'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
firebase.initializeApp(firebaseConfig)
class App extends Component {
constructor(props) {
super(props)
this.state = {
name: '',
city: '',
data: []
}
}
// componentDidMount(){
// firebase.database().ref('users').once('value')
// .then(snapshot =>{
// snapshot.forEach(item=>{
// this.state.data.push({
// id: item.key, ...item.val(),
// // name:this.state.name,
// // city:this.state.city
// })
// })
// })
// }
//
componentDidMount() {
firebase.database().ref('users').once('value')
.then(snapshot => {
snapshot.forEach(item => {
this.state.data.push({
id: item.key,
...item.val()
})
})
})
}
//push use for generate unique id and push the data to the Firebase
// componentDidMount(){
// firebase.database().ref('users').push({
// name: 'Sapan Kumar Das',
// city: 'Mumbai'
// })
// }
// handleSubmit =(e)=>{
// e.preventDefault()
// firebase.database().ref('users').child('01').set({
// name: this.state.name,
// city: this.state.city
// })
// }
// handleSubmit =(e)=>{
// // preventDefault just stop the Refesh!
// e.preventDefault()
// firebase.database().ref('users').child('02').set({
// name: this.state.name,
// city: this.state.city
// })
// }
//push for generate unique id
// Enter data to the Firebase
handleSubmit = (e) => {
e.preventDefault()
firebase.database().ref('users').push({
name: this.state.name,
city: this.state.city
})
}
render() {
return (
<>
<h1> Hello Firebase</h1>
{console.log(this.state.data)}
<form onSubmit={this.handleSubmit}>
<input type='text' placeholder='Enter Name' value={this.state.name} onChange={e => this.setState({ name: e.target.value })} />
<input type='text' placeholder='Enter City' value={this.state.city} onChange={e => this.setState({ city: e.target.value })} />
<input type='Submit' />
</form>
</>
)
}
}
export default App
import firebase from 'firebase'
const firebaseConfig = {
apiKey: "AIzaSyBSshtHVHQDZErWTOyZAZHFYrJ3TG-LbgU",
authDomain: "react-redux-firebase-f8847.firebaseapp.com",
databaseURL: "https://react-redux-firebase-f8847.firebaseio.com",
projectId: "react-redux-firebase-f8847",
storageBucket: "react-redux-firebase-f8847.appspot.com",
messagingSenderId: "437501214108",
appId: "1:437501214108:web:22cc6c4087caa9c3361c59"
};
firebase.initializeApp(firebaseConfig)
class App extends Component {
constructor(props) {
super(props)
this.state = {
name: '',
city: '',
data: []
}
}
// componentDidMount(){
// firebase.database().ref('users').once('value')
// .then(snapshot =>{
// snapshot.forEach(item=>{
// this.state.data.push({
// id: item.key, ...item.val(),
// // name:this.state.name,
// // city:this.state.city
// })
// })
// })
// }
//
componentDidMount() {
firebase.database().ref('users').once('value')
.then(snapshot => {
snapshot.forEach(item => {
this.state.data.push({
id: item.key,
...item.val()
})
})
})
}
//push use for generate unique id and push the data to the Firebase
// componentDidMount(){
// firebase.database().ref('users').push({
// name: 'Sapan Kumar Das',
// city: 'Mumbai'
// })
// }
// handleSubmit =(e)=>{
// e.preventDefault()
// firebase.database().ref('users').child('01').set({
// name: this.state.name,
// city: this.state.city
// })
// }
// handleSubmit =(e)=>{
// // preventDefault just stop the Refesh!
// e.preventDefault()
// firebase.database().ref('users').child('02').set({
// name: this.state.name,
// city: this.state.city
// })
// }
//push for generate unique id
// Enter data to the Firebase
handleSubmit = (e) => {
e.preventDefault()
firebase.database().ref('users').push({
name: this.state.name,
city: this.state.city
})
}
render() {
return (
<>
<h1> Hello Firebase</h1>
{console.log(this.state.data)}
<form onSubmit={this.handleSubmit}>
<input type='text' placeholder='Enter Name' value={this.state.name} onChange={e => this.setState({ name: e.target.value })} />
<input type='text' placeholder='Enter City' value={this.state.city} onChange={e => this.setState({ city: e.target.value })} />
<input type='Submit' />
</form>
</>
)
}
}
export default App
................................................................................................................................................................................................................................
React Native || Phone authentication
Step: #1
Step: #1
Generate a new project
Create a new React Native app by executing the following command in a terminal window:
react-native init PhoneAuth
Note npx helps us -
- NPX - A tool for executing Node packages.
- You don't want to install packages neither globally nor locally.
Step: #2
install following dependencies
yarn add react-native-firebase
Step: #3
Create a Firebase Project
visit the below the url
https://console.firebase.google.com/
Enter the Project name
Continue
Step: #4
Enable Phone Authentication
click on the Settings icon and go to Project settings
click on the button Add app, select the appropriate platform.
in mycase is Android
Step: #5 [Project] [fetch data from Firebase]
App.js
import React, { Component } from 'react';
import { StyleSheet, Text, View } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
componentDidMount() {
const myItems = Firebase.database().ref('/item');
myItems.on("value", datasnap => {
console.log(datasnap.val())
})
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
}
})
Happy Coding :)
.........................................................................................................................................................................................................................
Step: #6 [ Push or Insert into Firebase ]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
// componentDidMount() {
// const myItem = Firebase.database().ref('item');
// myItem.on("value", snapShot => {
// console.log(snapShot.val())
// })
// }
saveItem = () => {
//console.log(this.state.text)
const myWishes = Firebase.database().ref('mywish');
//data instert or push
myWishes.set({
text: this.state.text,
time: Date.now()
})
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })}
/>
<Button title='Submit' onPress={this.saveItem}></Button>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
..........................................................................................................................................................................................................................................
Step: #7 [ clear Text Fields and no overrides using push() ]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })}
/>
<Button title='Submit' onPress={this.saveItem}></Button>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #8 [ Display Data ] time <Text> {new Date (item.time).toDateString()}
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
componentDidMount() {
const myFetch = Firebase.database().ref('myDream');
myFetch.on("value", snapShot => {
// console.log(Object.values(snapShot.val()))
this.setState({ myList: Object.values(snapShot.val()) })
})
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
render() {
console.log(this.state)
const itemList = this.state.myList.map((item) => {
return (
<View>
<Text style={{fontSize:30}}> {item.text}</Text>
</View>
)
})
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })} />
<Button title='Submit' onPress={this.saveItem}></Button>
{itemList}
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #9 [ Remove]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
componentDidMount() {
const myFetch = Firebase.database().ref('myDream');
myFetch.on("value", snapShot => {
// console.log(Object.values(snapShot.val()))
//this.setState({ myList: Object.values(snapShot.val()) })
if (snapShot.val()) {
this.setState({ myList: Object.values(snapShot.val()) })
}
})
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
removeItem = () => {
Firebase.database().ref("myDream").remove();
// clear from display screen
this.setState({ myList: [] })
//this.setState({mylist:[{text:"removed successfully"}]})
}
render() {
console.log(this.state)
const itemList = this.state.myList.map((item) => {
return (
<View>
<Text style={{ fontSize: 30 }}> {item.text}</Text>
</View>
)
})
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })} />
<Button title='Submit' onPress={this.saveItem}></Button>
<View style={{ marginVertical: 10 }}>
<Button title='Delte' onPress={this.removeItem}></Button>
</View>
<View>
{itemList}
</View>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #10
Happy Coding :)
.............................................................................................................................................................................................................................................
Create a new React Native app by executing the following command in a terminal window:
react-native init PhoneAuth
Note npx helps us -
- NPX - A tool for executing Node packages.
- You don't want to install packages neither globally nor locally.
- Save time
Step: #2
install following dependencies
yarn add react-native-firebase
Step: #3
Create a Firebase Project
visit the below the url
https://console.firebase.google.com/
Enter the Project name
Continue
Choose it, Default account for Firebase and click the button create project
Continue
Step: #4
Enable Phone Authentication
click on the Settings icon and go to Project settings
click on the button Add app, select the appropriate platform.
in mycase is Android
Step: #5 [Project] [fetch data from Firebase]
App.js
import React, { Component } from 'react';
import { StyleSheet, Text, View } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
componentDidMount() {
const myItems = Firebase.database().ref('/item');
myItems.on("value", datasnap => {
console.log(datasnap.val())
})
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
}
})
Happy Coding :)
.........................................................................................................................................................................................................................
Step: #6 [ Push or Insert into Firebase ]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
// componentDidMount() {
// const myItem = Firebase.database().ref('item');
// myItem.on("value", snapShot => {
// console.log(snapShot.val())
// })
// }
saveItem = () => {
//console.log(this.state.text)
const myWishes = Firebase.database().ref('mywish');
//data instert or push
myWishes.set({
text: this.state.text,
time: Date.now()
})
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })}
/>
<Button title='Submit' onPress={this.saveItem}></Button>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
..........................................................................................................................................................................................................................................
Step: #7 [ clear Text Fields and no overrides using push() ]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
render() {
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })}
/>
<Button title='Submit' onPress={this.saveItem}></Button>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #8 [ Display Data ] time <Text> {new Date (item.time).toDateString()}
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
componentDidMount() {
const myFetch = Firebase.database().ref('myDream');
myFetch.on("value", snapShot => {
// console.log(Object.values(snapShot.val()))
this.setState({ myList: Object.values(snapShot.val()) })
})
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
render() {
console.log(this.state)
const itemList = this.state.myList.map((item) => {
return (
<View>
<Text style={{fontSize:30}}> {item.text}</Text>
</View>
)
})
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })} />
<Button title='Submit' onPress={this.saveItem}></Button>
{itemList}
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #9 [ Remove]
import React, { Component } from 'react';
import { StyleSheet, Text, View, TextInput, Button } from 'react-native';
import *as Firebase from 'firebase';
import { firebaseConfig } from './src/configs/firebaseConfig';
import Constants from 'expo-constants'
Firebase.initializeApp(firebaseConfig)
export default class App extends Component {
constructor(props) {
super(props)
this.state = {
text: '',
myList: []
}
}
componentDidMount() {
const myFetch = Firebase.database().ref('myDream');
myFetch.on("value", snapShot => {
// console.log(Object.values(snapShot.val()))
//this.setState({ myList: Object.values(snapShot.val()) })
if (snapShot.val()) {
this.setState({ myList: Object.values(snapShot.val()) })
}
})
}
saveItem = () => {
const myItem = Firebase.database().ref('myDream');
// No override using push()
myItem.push().set({
text: this.state.text
})
//clear text Fields
this.setState({ text: '' })
}
removeItem = () => {
Firebase.database().ref("myDream").remove();
// clear from display screen
this.setState({ myList: [] })
//this.setState({mylist:[{text:"removed successfully"}]})
}
render() {
console.log(this.state)
const itemList = this.state.myList.map((item) => {
return (
<View>
<Text style={{ fontSize: 30 }}> {item.text}</Text>
</View>
)
})
return (
<View style={styles.container}>
<Text> firebase Example</Text>
<TextInput style={styles.input}
value={this.state.text}
onChangeText={(text) => this.setState({ text })} />
<Button title='Submit' onPress={this.saveItem}></Button>
<View style={{ marginVertical: 10 }}>
<Button title='Delte' onPress={this.removeItem}></Button>
</View>
<View>
{itemList}
</View>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
// justifyContent: 'center',
paddingTop: Constants.statusBarHeight
},
input: {
borderColor: 'red',
borderWidth: 2
}
})
Happy Coding :)
.............................................................................................................................................................................................................................................
Step: #10
Happy Coding :)
.............................................................................................................................................................................................................................................
No comments:
Post a Comment