........................................................................................................................................................................................................................................
SafeArea
Expanded
Wrap
AnimatedContainer
Opacity
LayoutBuilder
PageView
Table
SliverAppBar
SliverList & SliverGrid
FadeInImage
StreamBuilder
InheritedModel
ClipRRect
Hero
CustomPaint
Tooltip
FittedBox
LayoutBuilder
.......................................................................................................................................................................................................................
1. How to Remove Debug Banner in Flutter?
When you create a new Flutter project for the first time you might have seen the Debug Banner on Right Top Corner of the application. Now a question arises that How to Remove Debug Banner in Flutter?
- Add debugShowCheckModeBanner: false in MaterialApp()
Widget and that should remove the banner on hot reload.
MaterialApp(
debugShowCheckedModeBanner: false
)
Note - The Debug Banner will also automatically be removed on a release build.
Loading
Tips #1
leading: InkWell(
child: Icon(Icons.menu),
onTap: () {
print("click menu");
},
),
Tips #2
iconTheme: IconThemeData(color: Colors.white, size: 20, opacity: 0.9),
Tips #3
textTheme
textTheme: TextTheme(title: TextStyle(color: Colors.white,fontSize: 30)),
Tips #4
brightness: Brightness.dark,
Tips #5
AppBar({
Key key,
this.leading,
this.automaticallyImplyLeading = true,
this.title,
this.actions,
this.flexibleSpace,
this.bottom,
this.elevation = 4.0,
this.backgroundColor,
this.brightness,
this.iconTheme,
this.textTheme,
this.primary = true,
this.centerTitle,
this.titleSpacing = NavigationToolbar.kMiddleSpacing,
this.toolbarOpacity = 1.0,
this.bottomOpacity = 1.0,
})
Tips #6[<]
automaticallyImplyLeading
If you don’t want the left-arrow on the app bar. you can use this parameter to hide it.automaticallyImplyLeading: false
Tips #7
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatelessWidget{
@override Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Home'),
bottom: PreferredSize(
child: Container(
alignment:Alignment.center,
color: Colors.green,
constraints: BoxConstraints.expand(height: 50),
child: Text('Hello'),
),
preferredSize: Size(50, 50),
),
),
body: Center(
),
);
}
}
Tips #8[How to fix bottom overflowed when keyboard shows error in Flutter?]
Below two way resolved the problem, but page will not be scroll
so my choice is SingleChildScrollView.
body: Center(
child: SinglechildScrollView(
...//
)
);
Note - We can do one at a time, don't mix it
resizeToAvoidBottomPadding: false,
resizeToAvoidBottomPadding: false,
resizeToAvoidBottomInset: false,
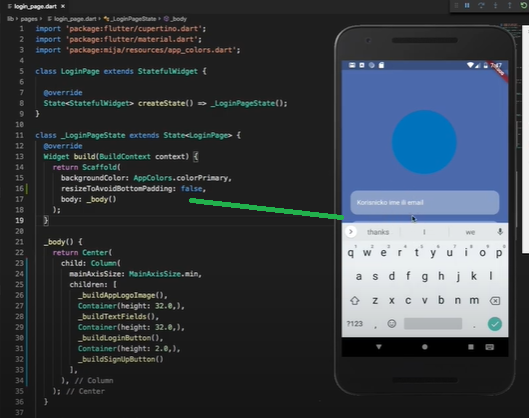
or
How to fix bottom overflowed when keyboard shows error in Flutter?
Scaffold(
resizeToAvoidBottomInset:false
);
or
body: Center(
child: SinglechildScrollView(
)
);
Tips #9
Tips #10
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
.......................................................................................................................................................................................................................................
How to change Status Bar Color
Only Android
void main() {
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle(
systemNavigationBarColor: Colors.blue, // navigation bar color
statusBarColor: Colors.pink, // status bar color
));
}
or
void main() async {
SystemChrome.setSystemUIOverlayStyle(
SystemUiOverlayStyle(statusBarColor: Colors.red));
runApp(MaterialApp(
home: MyApp(title: 'Hello Word'),
));
}
Both iOS and Android:
appBar: AppBar(
backgroundColor: Colors.red, // status bar color
brightness: Brightness.light, // status bar brightness
)
To apply for all app bars:
return MaterialApp(
theme: Theme.of(context).copyWith(
appBarTheme: Theme.of(context)
.appBarTheme
.copyWith(brightness: Brightness.light),
...
),
Hide Status bar
void main() {
SystemChrome.setEnabledSystemUIOverlays([SystemUiOverlay.bottom]);
runApp(MaterialApp(
debugShowCheckedModeBanner: false,
home: SplashScreen()));
}
You can add the below code in your main function to hide status bar.
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
));
...............
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
));
For single page
@override
void initState() {
SystemChrome.setEnabledSystemUIOverlays([SystemUiOverlay.bottom]);
super.initState();
}
@override
void dispose() {
SystemChrome.setEnabledSystemUIOverlays(
[SystemUiOverlay.top, SystemUiOverlay.bottom]);
super.dispose();
}
.......................................................................................................................................................................................................................................
Space-around vs space-evenly
Items spread across the screen however keeps equal space in
between (Space between)
items are evenly distributed in the line with equal space
around them (space around).
.......................................................................................................................................................................................................................................
How to Setup Height of the AppBar in Flutter ?
appBar: PreferredSize(
preferredSize: Size.fromHeight(100.0),
child: AppBar(
automaticallyImplyLeading: false, // hides leading widget
title:Text('Hello world'),
)
),
.........................................................................................................................................................................................................................
Example :#2[ Flutter AppBar Design]
import 'package:flutter/material.dart';
void main() {
runApp(new MyApp());
}
class MyApp extends StatelessWidget {
@override Widget build(BuildContext context) {
return new MaterialApp(
title: 'Generated App',
theme: new ThemeData(
primarySwatch: Colors.blue,
primaryColor: const Color(0xFF2196f3),
accentColor: const Color(0xFF2196f3),
canvasColor: const Color(0xFFfafafa),
),
home: new MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override _MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State {
@override Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
iconTheme: IconThemeData(color: Colors.white, size: 20, opacity: 0.9),
title: new Text('App Name'),
actions: [
// action button
Ink(
child: IconButton(
icon: Icon( Icons.search ),
onPressed: () { },
),
),
Ink(
child: IconButton(
icon: Icon( Icons.search ),
onPressed: () { },
),
),
],
leading: IconButton(
icon: Image.asset('assets/logo.png'),
onPressed: () { },
),
),
);
}
}
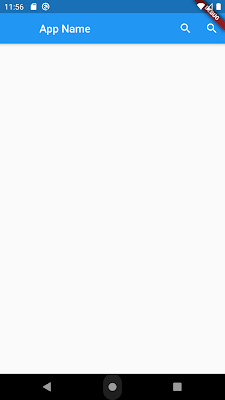
Now, we will add Leading and Action in setting icon in an AppBar Widget so our updated App bar widget will look like below.
appBar: AppBar(
leading: IconButton(
tooltip: 'Leading Icon',
icon: const Icon(
Icons.arrow_back,
),
onPressed: () {
// To do
},
),
title: const Text(
'Categories',
),
actions: <Widget>[
IconButton(
tooltip: 'Action Icon',
icon: const Icon(
Icons.settings,
),
onPressed: () {
// To do
},
),
],
),
AppBar with centered Title and Subtitle
AppBar(
automaticallyImplyLeading: false,
title: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Title",
style: TextStyle(fontSize: 18.0),
),
Text(
"subtitle",
style: TextStyle(fontSize: 14.0),
),
],
),
),
),
AppBar with Text and Icon Themes
AppBar(
backgroundColor: Colors.blueAccent,
title: Text("Title"),
actions: [
IconButton(
icon: Icon(Icons.search),
onPressed: () {},
),
],
iconTheme: IconThemeData(
color: Colors.white,
),
textTheme: TextTheme(
title: TextStyle(
color: Colors.white,
fontSize: 20.0
),
),
),
.................................................
import 'package:flutter/material.dart';
class HomeScreen extends StatelessWidget {
const HomeScreen() : super();
static const String title = 'Home';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(title),
),
body: Container(
child: Column(
children: [
],
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#3 [ Flutter AppBar and Search Bar]
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
bool isSearching = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.orange,
title: !isSearching
? Text('Home')
: TextField(
decoration: InputDecoration(
hintText: 'Search here',hintStyle: TextStyle(color: Colors.white,fontWeight: FontWeight.bold),
icon: Icon(Icons.search, color: Colors.white)),
),
actions: <Widget>[
isSearching
? IconButton(
onPressed: () {
setState(() {
this.isSearching = false;
});
},
icon: Icon(
Icons.cancel,
size: 22,
color: Colors.white,
),
)
: IconButton(
onPressed: () {
setState(() {
this.isSearching = true;
});
},
icon: Icon(
Icons.search,
size: 22,
color: Colors.white,
),
)
],
),
body: Center(),
);
}
}
.........................................................................................................................................................................................................................
Example :#4 [ Flutter Basic AppBar Design]
import 'package:flutter/material.dart';
void main() {
runApp(new MyApp());
}
class MyApp extends StatelessWidget {
@override Widget build(BuildContext context) {
return new MaterialApp(
title: 'Generated App',
theme: new ThemeData(
primarySwatch: Colors.blue,
primaryColor: const Color(0xFF2196f3),
accentColor: const Color(0xFF2196f3),
canvasColor: const Color(0xFFfafafa),
),
home: new MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override _MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State {
@override Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: new Text('App Name'),
actions: [
// action button
IconButton(
icon: Icon( Icons.search ),
onPressed: () { },
),
],
leading: IconButton(
icon: Image.asset('assets/logo.png'),
onPressed: () { },
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#5 [Flutter AppBar]
import 'package:flutter/material.dart';
void main() {
runApp(new MyApp());
}class MyApp extends StatelessWidget {
@override Widget build(BuildContext context) {
return new MaterialApp(
title: 'Generated App',
theme: new ThemeData(
primarySwatch: Colors.blue,
primaryColor: const Color(0xFF2196f3),
accentColor: const Color(0xFF2196f3),
canvasColor: const Color(0xFFfafafa),
),
home: new MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override _MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State {
@override Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: new Text('App Name'),
actions: <Widget>[
InkWell(
child: Icon(Icons.search),
onTap: () {
print("click search");
},
),
SizedBox(width: 20),
InkWell(
child: Icon(Icons.more_vert),
onTap: () {
print("click more");
},
),
SizedBox(width: 30) ],
leading: InkWell(
child: Icon(Icons.menu),
onTap: (){
print("click menu");
},
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#6 [ Default Tab Controller]
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
MyApp({Key key, this.title}) : super(key: key);
final String title;
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
@override Widget build(BuildContext context) {
return DefaultTabController(
length: 2,
child: Scaffold(
appBar: AppBar(
title: Text('Cart Applications!'),
centerTitle: true,
bottom: TabBar(
tabs: <Widget>[
Tab(text:'Product'),
Tab(text: 'Checkout',),
],
),
),
),
);
}
}
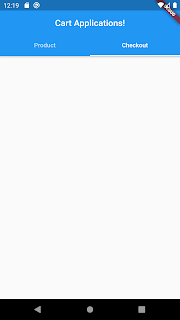
.........................................................................................................................................................................................................................
Example :#7 [Form Desing]
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Form Validation'),
centerTitle: true,
),
body: Container(
padding: EdgeInsets.all(30.0),
child: Column(
children: <Widget>[
ProductName(),
ProductPrice(),
SaveButton(),
],
),
),
);
}
}
Widget ProductName() {
return TextField(
decoration: InputDecoration(
labelText: 'Enter username',
),
);
}
Widget ProductPrice() {
return TextField(
decoration: InputDecoration(
labelText: 'Enter price'),
);
}
Widget SaveButton() {
return RaisedButton(
child: Text('Save Products'),
onPressed: () {}
,
);
}
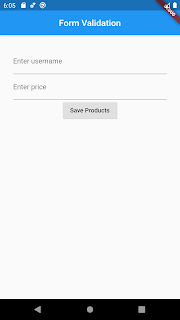
.........................................................................................................................................................................................................................
Example :#8 [Buttons with Icons]
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatefulWidget{
@override MyAppState createState()=>MyAppState();
}
class MyAppState extends State<MyApp>{
@override Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Image'),
),
body: Center(
child: RaisedButton.icon(
onPressed: (){},
icon: Icon(Icons.mail),
label: Text('mail Box'),
color: Colors.amber,
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#9 [Expanded Widget ]
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatefulWidget{
@override MyAppState createState()=>MyAppState();
}
class MyAppState extends State<MyApp>{
@override Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Image'),
),
body: Row(
children: <Widget>[
Expanded(
flex: 3,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.amber,
child: Text('1'),
),
),
Expanded(
flex: 2,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.green,
child: Text('2'),
),
),
Expanded(
flex: 1,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.red,
child: Text('3'),
),
),
],
),
);
}
}
.........................................................................................................................................................................................................................
Example :#10 [Expanded Widget ]
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatefulWidget{
@override MyAppState createState()=>MyAppState();
}
class MyAppState extends State<MyApp>{
@override Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
title: Text('Image'),
),
body: Row(
children: <Widget>[
Expanded(
flex: 3,
child: Image.asset('assets/images/onboarding1.png'),
),
Expanded(
flex: 3,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.amber,
child: Text('1'),
),
),
Expanded(
flex: 2,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.green,
child: Text('2'),
),
),
Expanded(
flex: 1,
child: Container(
padding: EdgeInsets.all(30.0),
color: Colors.red,
child: Text('3'),
),
),
],
),
);
}
}
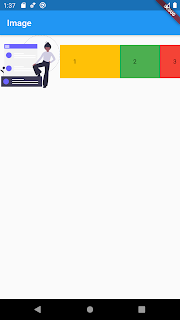
.........................................................................................................................................................................................................................
Example :#11 [List ]
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
MyApp({Key key}) : super(key: key);
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp>{
List<String> mylist=[
'ddd....',
'dddddddd',
'dddddddd',
'dddddddd',
'dddddddd',
];
@override
Widget build(BuildContext context ){
return Scaffold(
appBar: AppBar(
title: Text('App Bar '),
),
body: Container(
child: Column(
children: mylist.map((e) => Text(e)).toList()
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#12 [ List ]
class Quotes {
String title;
String author;
Quotes({this.title, this.author});
}
import 'package:flutter/material.dart';
import 'package:helloworld/list.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
MyApp({Key key}) : super(key: key);
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp>{
List<Quotes> mylist= [
Quotes(title:'Hello', author:'Peetor'),
Quotes(title:'Hello', author:'Hary'),
];
@override
Widget build(BuildContext context ){
return Scaffold(
appBar: AppBar(
title: Text('App Bar'),
),
body: Container(
child: Column(
children: mylist.map((e) =>Text('${e.title}' '${e.author}')).toList(),
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#13 [Card]
class Quotes {
String title;
String author;
Quotes({this.title, this.author});
}
import 'package:flutter/material.dart';
import 'package:helloworld/list.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
MyApp({Key key}) : super(key: key);
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
List<Quotes> e = [
Quotes(title: 'Hello...........................Hello...........................', author: 'Peetor.....'),
Quotes(title: 'Hello.....Hello..............................................', author: 'Hary..........'),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('App Bar'),
),
body: Container(
child: Column(
children:
e.map((e) => quoteCard(e)).toList(),
),
),
);
}
}
Widget quoteCard(e) {
return Card(
margin: EdgeInsets.fromLTRB(16.0, 16.0, 16.0, 0),
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Text(
e.title,
style: TextStyle(fontSize: 20.0, color: Colors.grey[800]),
),
SizedBox(
height: 20.0,
),
Text(
e.author,
style: TextStyle(fontSize: 20.0, color: Colors.grey[800]),
),
SizedBox(
height: 20.0,
),
],
),
),
);
}
.........................................................................................................................................................................................................................
Example :#14 [Loading]
flutter_spinkit 4.1.2+1
.........................................................................................................................................................................................................................
Example :#15 [AppBar ]
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatefulWidget{
@override MyAppState createState()=>MyAppState();
}
class MyAppState extends State<MyApp>{
@override Widget build(BuildContext context){
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.orange[400],
shape: RoundedRectangleBorder(borderRadius: BorderRadius.vertical(
bottom: Radius.circular(30),
)),
),
);
}
}
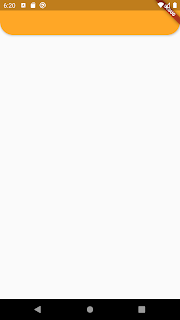
.........................................................................................................................................................................................................................
Example :#16 [Shopping header Desing]
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
));
class MyApp extends StatefulWidget {
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView(
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Padding(
padding: EdgeInsets.only(top: 15.0),
child: IconButton(icon: Icon(Icons.arrow_back)),
),
Padding(
padding: EdgeInsets.only(top: 15.0, right: 15.0),
child: Stack(
children: <Widget>[
Container(
width: 50,
height: 50,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20)),
),
Container(
width: 40,
height: 40,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
color: Colors.green[900]),
child: Icon(
Icons.shopping_basket,
size: 20.0,
color: Colors.white,
),
),
Positioned(
top: 25.0,
right: 30.0,
child: Container(
width: 20,
height: 20,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
color: Colors.red[900]),
child: Center(
child: Text(
'8',
style: TextStyle(color: Colors.white),
),
),
),
)
],
),
)
],
)
],
),
);
}
}
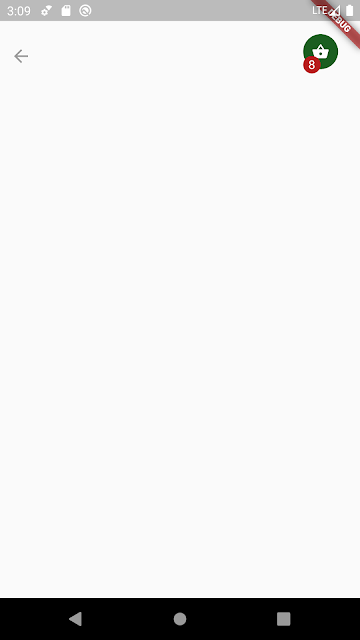
.........................................................................................................................................................................................................................
Example :# 17
import 'package:flutter/material.dart';
void main()=>runApp(MaterialApp(home: MyApp(),));
class MyApp extends StatefulWidget{
@override
MyAppState createState()=>MyAppState();
}
class MyAppState extends State<MyApp>{
@override
Widget build(BuildContext context){
return Scaffold(
body:Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
TextSection(Colors.yellowAccent),
TextSection(Colors.green),
TextSection(Colors.deepPurpleAccent),
],
) ,
);
}
}
class TextSection extends StatelessWidget{
Color _colors;
TextSection(this._colors);
@override
Widget build(BuildContext context){
return Container(
decoration: BoxDecoration(color: _colors),
child: Text('Hello World', style: TextStyle(fontSize: 20.0),),
);
}
}
.........................................................................................................................................................................................................................
Example :#18
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SignInPage(),
);
}
}
class SignInPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Time Picker'),
centerTitle: true,
backgroundColor: Colors.grey,
),
body: contextBuilder(),
);
}
}
class contextBuilder extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Padding(
padding: EdgeInsets.all(20.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Text(
'Sign In',
style: TextStyle(fontSize: 28.0, fontWeight: FontWeight.bold),
textAlign: TextAlign.center,
),
RaisedButton(
onPressed: () {},
child: Text(
'Sign in with Google',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 18.0,
color: Colors.black),
),
color: Colors.white,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0))),
),
SizedBox(
height: 8.0,
),
SignInButton(
onPressed: () {},
borderRadius: 4.0,
color: Colors.white,
child: Text(
'Sign in with Facebook',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 18.0,
color: Colors.black),
),
),
SizedBox(height: 4.0),
RaisedAbstract(
text: 'Sign in with Twitter',
textColor: Colors.black,
color: Colors.teal[700],
onPressed: () {},
),
],
),
);
}
}
class RaisedAbstract extends SignInButton {
RaisedAbstract({
Color color,
String text,
Color textColor,
double borderRadius,
VoidCallback onPressed,
}) : super(
child: Text(
text,
style: TextStyle(
color: textColor, fontSize: 18.0, fontWeight: FontWeight.bold),
),
color: color,
borderRadius: 8.0,
onPressed: onPressed,
height: 8.0,
);
}
class SignInButton extends StatelessWidget {
SignInButton({
this.child,
this.color,
this.borderRadius,
this.onPressed,
this.height: 50,
});
final Widget child;
final Color color;
final double borderRadius;
final double height;
final VoidCallback onPressed;
@override
Widget build(BuildContext context) {
return SizedBox(
height: 50.0,
child: RaisedButton(
child: child,
color: color,
onPressed: onPressed,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(borderRadius))),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#19
import 'package:flutter/material.dart';
void main()=>runApp(MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
return MaterialApp(
debugShowCheckedModeBanner: false,
home: FirstScreen(),
);
}
}
class FirstScreen extends StatefulWidget{
@override
FirstScreenState createState()=>FirstScreenState();
}
class FirstScreenState extends State<FirstScreen>{
@override
Widget build(BuildContext context){
return Scaffold(
body: ListView(
children: <Widget>[
Padding(
padding: EdgeInsets.all(15.0),
child: Row(
children: <Widget>[
Icon(Icons.menu, color: Colors.black),
Padding(
padding: EdgeInsets.all(15.0),
child: Container(
height: 50,
width: 50,
decoration: BoxDecoration(
boxShadow: [
BoxShadow( color: Colors.grey.withOpacity(1.0),
blurRadius: 4.0,
spreadRadius: 5.0,
offset: Offset(0.2, 0.3),)
],
shape: BoxShape.circle,
image: DecorationImage(
image: AssetImage('assets/images/l.jpg'),
)
),
),
)
],
),
)
],
),
);
}
}
.........................................................................................................................................................................................................................
Example :#20
import 'package:flutter/material.dart';
import 'package:shoppingproject/screens/homeScreens.dart';
void main()=>runApp(MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
return MaterialApp(
title:'Flutter App',
debugShowCheckedModeBanner: false,
home:HomeScreen() ,
theme: ThemeData(
primaryColor: Colors.red,
accentColor: Color(0xFFFE9ED),
),
);
}
}
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget{
@override
HomeScreenState createState()=>HomeScreenState();
}
class HomeScreenState extends State<HomeScreen>{
@override
Widget build(BuildContext context){
return Scaffold(
backgroundColor: Theme.of(context).primaryColor,
appBar: AppBar(
title: Text('Chat', style: TextStyle(fontSize: 22, fontWeight: FontWeight.bold)),
centerTitle: true,
leading: IconButton(
onPressed: (){},
icon: Icon(Icons.menu),
iconSize: 30.0,
color: Colors.white,
),
actions: <Widget>[
InkWell(
child: IconButton(
onPressed: (){},
icon: Icon(Icons.more_horiz),
iconSize: 25,
color: Colors.white,
),
),
],
),
body: Column(
children: <Widget>[
CategorySelector(),
Expanded(
child: Container(
decoration:BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.only(
topRight: Radius.circular(30.0),
topLeft: Radius.circular(30.0),
)
),
),
)
],
),
);
}
}
class CategorySelector extends StatefulWidget{
@override
CategorySelectorState createState()=>CategorySelectorState();
}
class CategorySelectorState extends State<CategorySelector>{
int selectIndex =0;
final List<String> category =['Message', 'Online', 'Groups','Chats', 'Request'];
@override
Widget build(BuildContext context){
return Container(
height: 90.0,
color: Theme.of(context).primaryColor,
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: category.length,
itemBuilder: (context, int index){
return GestureDetector(
onTap: (){
setState(() {
selectIndex=index;
});
},
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 20.0, vertical: 30.0),
child: Text(category[index],
style: TextStyle(color: index == selectIndex ?Colors.white: Colors.white60, fontWeight: FontWeight.bold, fontSize: 20.0, letterSpacing: 1.2),),
),
);
}),
);
}
}
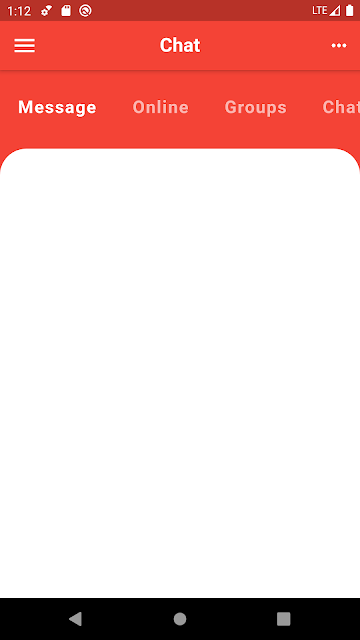
.........................................................................................................................................................................................................................
Example :#21
.........................................................................................................................................................................................................................
Example :#22 [Custom Widget]
import 'package:flutter/material.dart';
void main()=> runApp(MaterialApp(home: CustomWidgetDemo()));
class CustomWidgetDemo extends StatefulWidget{
CustomWidgetDemo({Key key}):super(key:key);
final String title="Custom Widgets";
@override
_CustomWidgetDemoState createState()=>_CustomWidgetDemoState();
}
class _CustomWidgetDemoState extends State<CustomWidgetDemo> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Text("here is a custom button in FLutter"),
CustomButton(
onPressed: () {
print("Tapped Me");
},
)
],
),
),
);
}
}
class CustomButton extends StatelessWidget {
CustomButton({@required this.onPressed});
final GestureTapCallback onPressed;
@override
Widget build(BuildContext context) {
return RawMaterialButton(
fillColor: Colors.green,
splashColor: Colors.greenAccent,
onPressed: onPressed,
shape: StadiumBorder(),
child: Padding(
padding: EdgeInsets.all(10.0),
child: Row(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
Icon(
Icons.face,
color: Colors.amber,
),
SizedBox(
width: 10.0,
),
Text(
"Tap Me",
maxLines: 1,
style: TextStyle(color: Colors.white),
),
],
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#23
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(home: MyApp()));
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: SingleChildScrollView(
child: Container(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
SizedBox(
height: 50,
),
/// Search Bar
Container(
margin: EdgeInsets.symmetric(horizontal: 12),
padding: EdgeInsets.symmetric(horizontal: 16, vertical: 14),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(8),
boxShadow: <BoxShadow>[
BoxShadow(
offset: Offset(5.0, 5.0),
blurRadius: 5.0,
color: Colors.black87.withOpacity(0.05),
),
],
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Padding(
padding: const EdgeInsets.only(left: 9),
child: Text(
"Search",
style: TextStyle(color: Color(0xff9B9B9B), fontSize: 17),
),
),
Spacer(),
Icon(Icons.search),
],
),
),
]))));
}
}
.........................................................................................................................................................................................................................
Example :#24
import 'package:flutter/material.dart';
void main()=>runApp(MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Shopping(),
);
}
}
class Shopping extends StatefulWidget{
@override
ShoppingState createState()=>ShoppingState();
}
class ShoppingState extends State<Shopping>{
@override
Widget build(BuildContext context){
return Scaffold(
backgroundColor: Color(0xff4E2958),
body: SingleChildScrollView(
child: SafeArea(
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 12),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
IconButton(color: Colors.white,onPressed: (){},icon: Icon(Icons.arrow_back_ios),),
IconButton(color: Colors.white,onPressed: (){},icon: Icon(Icons.search),)
],
),
),
SizedBox(height: 30),
Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.vertical(top: Radius.circular(30)),
),
),
],
),
),
),
);
}
}
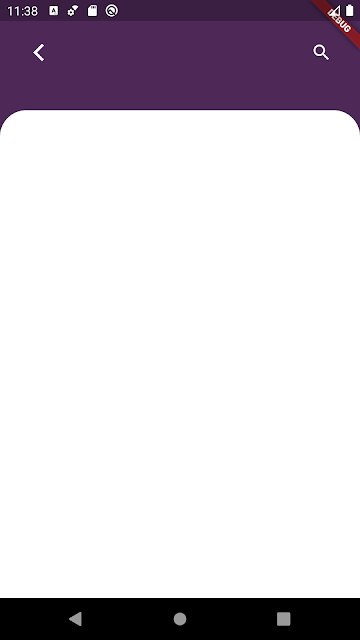
.........................................................................................................................................................................................................................
Example :#25
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(home: Home()));
class Home extends StatefulWidget {
@override
HomeState createState() => HomeState();
}
class HomeState extends State<Home> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
elevation: 0.0,
backgroundColor: Colors.transparent,
iconTheme: IconThemeData(color: Colors.black),
leading: IconButton(
onPressed: () {},
icon: Icon(Icons.format_quote,size: 50,),
),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Text(
'Anyone who has never made a mistake has never tried anything new.',
style: Theme.of(context)
.textTheme
.headline3
.copyWith(color: Colors.grey.shade800)),
SizedBox(
height: 12.0,
),
Text('Albert Einstein',
style: Theme.of(context)
.textTheme
.subtitle1
.copyWith(color: Colors.grey.shade600, fontSize: 20.0))
]),
),
bottomNavigationBar: BottomAppBar(
child: Row(
children: [
const SizedBox(width: 20,),
Expanded(
child: GestureDetector(
onTap: () {},
child: Text('tap for more', textAlign: TextAlign.center,),
)),
IconButton(
onPressed: () {},
icon: Icon(Icons.bookmark_border),
),
IconButton(
onPressed: () {},
icon: Icon(Icons.share),
)
],
),
),
);
}
}
.......................................................................................................................................................................................................................................
Example :#26 [ Exploring Packages shape_of_view 1.0.2]
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:shape_of_view/shape_of_view.dart';
void main() async {
SystemChrome.setSystemUIOverlayStyle(
SystemUiOverlayStyle());
runApp(MaterialApp(
debugShowCheckedModeBanner: false,
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Color(0xFFEDF3F8),
body: ListView(
children: [
ShapeOfView(
shape: ArcShape(height: 60, position: ArcPosition.Bottom),
child: Image.asset(
'assets/images/beach.jpg',
height: 150,
fit: BoxFit.cover,
),
),
SizedBox(
height: 40,
),
Center(
child: Text("Hotel ",
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 35,
color: Color(0xFFF22547E))),
),
SizedBox(height: 60),
Padding(
padding: EdgeInsets.only(right: 40, left: 40),
child: RoundedButton(
hinText: 'Email',
prefixIcon: Icon(Icons.email),
),
),
SizedBox(height: 30),
Padding(
padding: EdgeInsets.only(right: 40, left: 40),
child: RoundedButton(
hinText: 'password',
prefixIcon: Icon(Icons.lock),
),
),
SizedBox(height: 30),
FlatButton(
onPressed: () {},
child: Container(
padding: EdgeInsets.fromLTRB(60.0, 16.0, 60.0, 16.0),
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blue[900], Colors.blue[200]]),
borderRadius: BorderRadius.circular(50.0),
),
child: Text(
'Sign In',
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 24),
),
),
),
SizedBox(height: 30),
FlatButton(
onPressed: () {},
child: Container(
padding: EdgeInsets.fromLTRB(60.0, 16.0, 60.0, 16.0),
child: Text("Don't you have an account ? Sign up",
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 16,
color: Color(0xFF54B0F3)))),
),
],
),
);
}
}
class RoundedButton extends StatelessWidget {
final String hinText;
final prefixIcon;
RoundedButton({Key key, @required this.hinText, this.prefixIcon}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
padding: const EdgeInsets.all(8.0),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(50),
),
child: TextField(
decoration: InputDecoration(
contentPadding: const EdgeInsets.all(12.0),
hintText: hinText,
prefixIcon: prefixIcon,
hintStyle: TextStyle(color: Color(0xFF54B0F3)),
border: InputBorder.none),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#27 [Basic Steps ]
colors
image
.........................................
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
);
}
}
class Home extends StatefulWidget {
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Hello'),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
TextSctions(Colors.red),
TextSctions(Colors.black),
TextSctions(Colors.blueAccent),
],
),
);
}
}
class TextSctions extends StatelessWidget {
TextSctions(this._color);
final Color _color;
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
color: _color,
),
child: Text('Hi'),
);
}
}
........................................................
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
);
}
}
class Home extends StatefulWidget {
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('hello'),
),
body: Column(
children: [
ImageBaner('assets/images/d.jpg'),
TextSection(Colors.red),
TextSection(Colors.green),
],
),
);
}
}
class ImageBaner extends StatelessWidget {
ImageBaner(this.assetsPath);
final assetsPath;
@override
Widget build(BuildContext context) {
return Container(
constraints: BoxConstraints.expand(
height: 200,
),
decoration: BoxDecoration(color: Colors.white),
child: Image.asset(assetsPath, fit: BoxFit.cover),
);
}
}
class TextSection extends StatelessWidget {
final Color _color;
TextSection(this._color);
@override
Widget build(BuildContext context) {
return Container(
color: _color,
child: Text('Hello'),
constraints: BoxConstraints.expand(
height: 100,
),
);
}
}
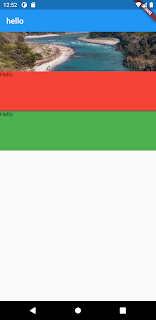
.........................................................................................................................................................................................................................
Example :#28
import 'dart:convert';
import 'package:http/http.dart' as http;
import 'package:flutter/material.dart';
import 'package:price_comparing_app/custombuttons/signinbutton.dart';
import 'package:price_comparing_app/signin.dart';
class SignUp extends StatefulWidget {
static const String SignUpScreenRoute = 'SignUpScreen';
@override
_SignUpState createState() => _SignUpState();
}
class _SignUpState extends State<SignUp> {
//we use this variable with state to show and hide circular indicator
bool visible = false;
final nameController = TextEditingController();
final emailController = TextEditingController();
final passwordController = TextEditingController();
final confirmpasswordController = TextEditingController();
Future userRegistration() async {
//Showing circular indicator
setState(() {
visible = true;
});
// SERVER API URL
var url =
'http://weanio.com/portfolio/price_comparing/public/api/auth/signup';
//for data storing
var data = {
'name': nameController.text,
'email': emailController.text,
'password': passwordController.text,
'confirmpassword': ""
};
var response = await http.post(url, body: json.encode(data));
// var response = await http.post(url, body: data);
// Getting server response into variable
var message = jsonDecode(response.body);
Navigator.push(context,
MaterialPageRoute(builder: (BuildContext context) => SignIn()));
// print(nameController.text);
// print(confirmpasswordController.text);
// If web call success than hide the CirculrProgressIndicator
if (response.statusCode == 200) {
setState(() {
visible = false;
});
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text(message),
actions: [
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('OK')),
],
);
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: SingleChildScrollView(
child: Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
padding: EdgeInsets.only(
top: MediaQuery.of(context).size.height * .03,
bottom: MediaQuery.of(context).size.height * .02),
decoration: BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: AssetImage(
'images/bg.png',
))),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Padding(
padding: const EdgeInsets.only(top: 0),
child: Image.asset(
'images/spara.png',
height: 60,
),
),
Padding(
padding: const EdgeInsets.only(left: 20, right: 20),
child: Card(
elevation: 30,
color: Colors.white,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10)),
child: Container(
decoration:
BoxDecoration(borderRadius: BorderRadius.circular(10)),
width: 390,
height: 500,
child: Padding(
padding: const EdgeInsets.all(15),
child: Column(
children: [
Text(
'Create Account',
style: TextStyle(
color: Colors.black,
fontSize: 20,
),
),
SizedBox(
height: 15,
),
TextField(
controller: nameController,
decoration: InputDecoration(
labelText: 'User Name',
suffixIcon: Icon(Icons.person),
hintText: 'Enter Your Name'),
),
SizedBox(
height: 10,
),
TextField(
controller: emailController,
decoration: InputDecoration(
labelText: 'Email',
suffixIcon: Icon(Icons.person),
hintText: 'Enter your Email'),
),
SizedBox(
height: 15,
),
TextField(
controller: passwordController,
decoration: InputDecoration(
labelText: 'Password',
suffixIcon: Icon(Icons.visibility),
hintText: 'Enter Your password'),
autofocus: false,
obscureText: true,
),
TextField(
controller: confirmpasswordController,
decoration: InputDecoration(
labelText: 'Confirm Password',
suffixIcon: Icon(Icons.visibility),
hintText: 'Enter Your Confirm Password'),
autofocus: false,
obscureText: true,
),
SizedBox(
height: 10,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Checkbox(
checkColor: Colors.green,
activeColor: Colors.grey,
value: true,
onChanged: (value) {}),
Text(
'I read and agreeto ',
style: TextStyle(color: Colors.grey),
),
Text(
'Terms&Conditions',
style: TextStyle(
decoration: TextDecoration.underline,
color: Colors.red,
),
)
],
),
SizedBox(
height: 0,
),
SignInButton(
text: 'SIGNUP',
textColor: Colors.white,
color: Colors.lime[300],
onPressed: () {
userRegistration();
},
),
Visibility(
visible: visible,
child: Container(
margin: EdgeInsets.only(bottom: 30),
child: CircularProgressIndicator(),
),
),
SizedBox(
height: 2,
),
Text('or Login using social media'),
SizedBox(
height: 15,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Image.asset(
'images/facebook.png',
height: 28,
width: 23,
),
SizedBox(
width: 35,
),
Image.asset(
'images/instagram.png',
height: 30,
width: 30,
),
SizedBox(
width: 35,
),
Image.asset(
'images/twitter.png',
height: 30,
width: 30,
),
],
)
],
),
),
),
),
),
SizedBox(
height: 0,
),
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(
text: 'Have an account',
style: TextStyle(color: Colors.black, fontSize: 15)),
TextSpan(
text: ' SIGN IN here ',
style: TextStyle(color: Colors.red, fontSize: 15)),
],
),
),
],
),
),
),
);
}
}
.........................................................................................................................................................................................................................
Example :#29
.........................................................................................................................................................................................................................
Example :#30